Bash if else Statement
Bash if statement, if else statement, if elif else statement and nested if statement used to execute code based on a certain condition. Condition making statement is one of the most fundamental concepts of any computer programming. Moreover, the decision making statement is evaluating an expression and decide whether to perform an action or not.
In this tutorial, you will learn Bash if
statements and how to use them in your bash script. Moreover, we can understand if statements through the flow diagram.
The Bash if
statements represent the following 4 different type forms.
if
Statementif..else
Statementif..elif..else
Statement- Nested
if
Statements
Bash if
Statement #
The most basic form of if
statement followed by the then statement. The Bash if
statement writes in the following form:
if EXPRESSION
then
STATEMENTS
fi
If the EXPRESSION
evaluates to True
, the STATEMENTS
will execute. If the EXPRESSION
returns False
, execution will move to the next statement.
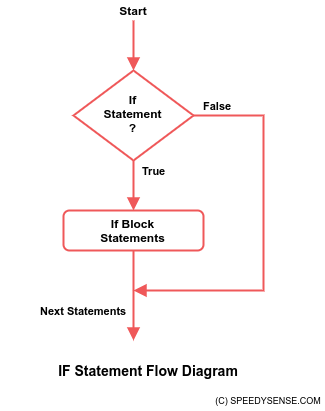
Let’s take a look at the following example, the check value is positive.
#!/bin/bash
echo -n "Enter a numeric value: "
read value
if [ $value -gt 0 ]
then
echo "Value is Positive"
fi
Save the above code in if_expr.sh
file and run it from the command line:
bash if_expr.sh
The above script will ask you to enter a number. You can enter any positive value. This will give you the following value if you enter any positive value.
Output
Value is Positive
Bash if..else
Statement #
The Bash if..else
statement writes in the following form:
if EXPRESSION
then
STATEMENTS1
else
STATEMENTS2
fi
If the EXPRESSION
evaluates to True
, the STATEMENTS1
will execute. If EXPRESSION
returns False
, the STATEMENTS2
will execute.
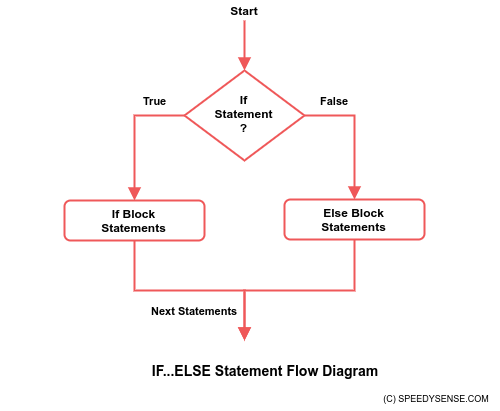
In the following example, we added else
clause in the previous example.
#!/bin/bash
echo -n "Enter a numeric value: "
read value
if [ $value -gt 0 ]
then
echo "Value is positive"
else
echo "Value is negative"
fi
Finally, the above example check value is positive or negative based on whatever value you enter.
Bash if..elif..else
Statement #
The else if
is used for multiple if
conditions. However, the else
clause is optional. The Bash if..elif..else
statement written the following form.
if EXPRESSION1
then
STATEMENTS1
elif EXPRESSION2
Then
STATEMENTS2
else
STATEMENTS3
fi
If the EXPRESSION1
evaluates to True
, the STATEMENTS1
will be executed. If the EXPRESSION2
evaluates to True
, the STATEMENTS2
will be executed. However, if all EXPRESSION
evaluates to False
, the STATEMENTS3
will be executed.
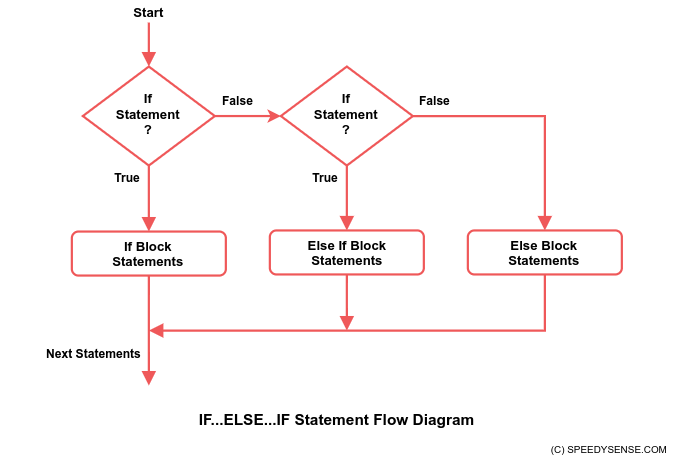
In our previous example, we added the elif
clause.
#!/bin/bash
echo -n "Enter a numeric value: "
read value
if [ $value -gt 0 ]
then
echo "Value is positive"
elif [ $value -eq 0 ]
then
echo "Value is 0"
else
echo "Value is negative"
fi
The above example check value is positive, negative, or equal to zero based on whatever value you enter.
Bash Nested if
Statements #
Lastly, bash allows you to place nested if
statement. You can place multiple if
statement within if
statement.
The following example will prompt you to enter numbers. The script will check value is positive or negative. Even more, check value is more than 10.
#!/bin/bash
echo -n "Enter a numeric value: "
read value
if [ $value -gt 0 ]
then
if [[ $value -ge 10 ]]
then
echo "Value is positive and greater than 10."
else
echo "Value is positive and less than 10."
fi
else
if [[ $value -le -10 ]]
then
echo "Value is negative and more than -10."
else
echo "Value is negative and less than -10."
fi
fi
Final Thoughts
That all you need to know how to if
, if..else
and if..elif..else
statements allow you to control the flow in Bash script based on evaluating the given conditions. Furthermore, we understand if statements through the flow diagram. Even more, we have seen four different if statement examples.
We hope you have found this article helpful. Let us know your questions or feedback if any through the comment section in below. You can subscribe our newsletter and get notified when we publish new WordPress articles for free. Moreover, you can explore here other interesting articles.
Note: Icon made by Pixelmeetup.
If you like our article, please consider buying a coffee for us.
Thanks for your support!
Buy me a coffee!
Join the Discussion.